Advanced Python 3 | Common Misunderstandings
Advanced Python 3 | Common Misunderstandings
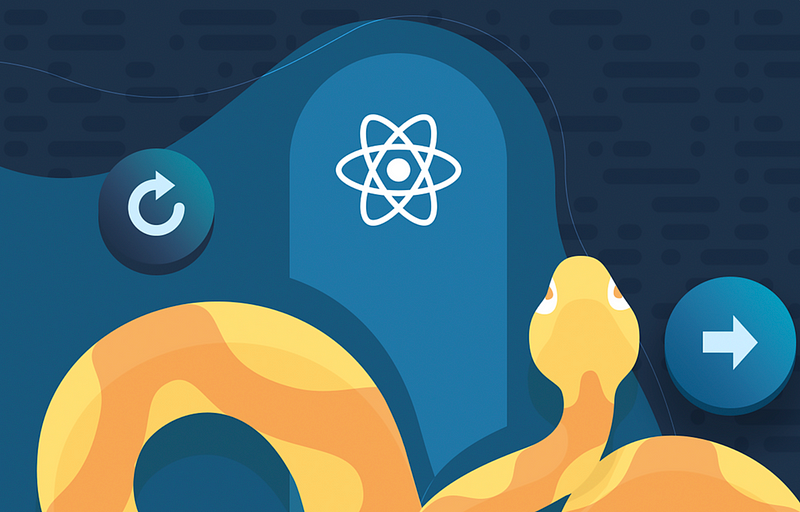
There are many simple and basic things in python that we have some mistakes on these kinds of stuff. This is a summary of common misunderstandings or misreadings of the python code.
- Identity #1: What is the result of the following code?
The answer is:
True
This is because, for simple int variables, it saves the memory that we assign the same memory to different variables if the values are the same. So, for different variables with the same value, they will have the same id.
2. Identity #2: What is the result of the following code?
The answer is:
False
This is because the floats are not simple data types.
3. Variable Name #1: What is the result of the following code?
The answer is:
2
_2 can be a variable name in python.
4. Variable Name #2: What is the result of the following code?
The answer is:
Error
$ can’t be in the variable name (as well as -), only _, letters (some foreign characters) or numbers (not in the first place) are valid (except for reserved keywords).
5. Strings: What is the result of the following code?
The answer is:
' *\n *** \n***** \n *** \n *\n *\n * '
If we directly print the strings, this will give us a single line with escape characters.
5. Indent: How long is the indent?
The answer is:
8 spaces
If we want to change it to 4 spaces, we have to write like,
print("\ta".expandtabs(tabsize=4))
6. Boolean #1: What is the result of the following code?
The answer is:
True
“False” values include:
- False
- None
- zero of any numeric type:
0, 0.0, 0j, Decimal(0), Fraction(0, 1)
- empty sequences and collections:
'', (), [], {}, set(), range(0)
7. Boolean #2: What is the result of the following code?
The answer is:
Error
This is because ‘none’ does not equal to ‘None’. If we change the code to,
bool(inf) == bool(not None)
then the answer will be,
True
“True” values include:
- True
- not None
- 1
inf
- 42 (and other values)
- ‘42’ (and other not empty sequences)
8. Scope #1: What is the result of the following code?
The answer is:
1
9. Scope #2: What is the result of the following code?
The answer is:
None
10. Float Sum-up: What is the result of the following code?
The answer is:
False
This is because the 0.1 + 0.2 is calculated to 0.30000000000000004
due to the Python interpreter.
11. Complex #1: What is the result of the following code?
The answer is:
(10.99+0j)