Advanced Python 4 | List Methods, Tuple Methods, List Comprehensions, Map, Lambda, Filter, and…
Advanced Python 4 | List Methods, Tuple Methods, List Comprehensions, Map, Lambda, Filter, and Reduce
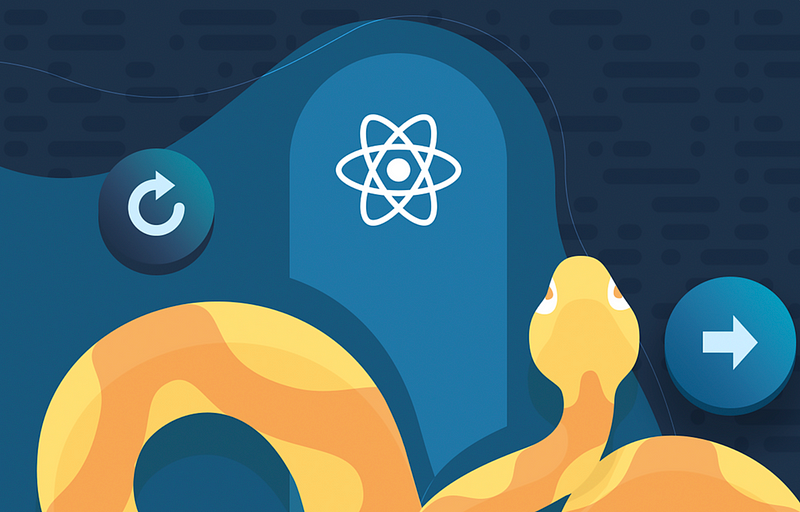
- Methods for Lists and Tuples
The dir()
function can give us all the attributes of a given class. For example, if we ignore all the attributes start with a '__’
sign (which means private attributes), then we can get a list of methods (public attributes) for any given class. We can use this to print all the methods of a list.
[method for method in dir(list) if not method.startswith('__')]
Then we can have,
['append',
'clear',
'copy',
'count',
'extend',
'index',
'insert',
'pop',
'remove',
'reverse',
'sort']
We can also do it to the tuple.
[method for method in dir(tuple) if not method.startswith('__')]
Then we can have,
['count', 'index']
We can also use tab after .
, then the compiler will give us a list of methods we can choose and use for a given object.
(1) In-place Method
For some of the methods we use, it will replace the original value with the return value without an assigning statement. This is called an in-place method.
(2) Sort Method
The .sort()
method will return a sorted list. This method is an in-place method so that we don’t have to assign it back. The list is sorted in the following sequence:
- For numbers: sort from negative numbers to positive numbers
- For characters: sort from number chars, then from A to z
- For string: Follow the same sorting query of the characters
- We can not mix data types for sorting (characters and strings can be mixed for sorting), and also, we can not sort for data types expect for numbers, characters, and string.
Note that if we want to reverse the result, we can set the reverse
parameter to True
.
- Example: What is the output of the following program?
Output:
None
This is because the sort method is an in-place method so it returns None as it’s value.
(3) Append and Extend Method
The .append()
method will put a new element in the last of the list, while the .extend()
method will extend the list with a given sequence. This method is an in-place method so that we don’t have to assign it back.
- Example #1. What is the output of the following program?
Output:
Error
This is because + operator can only concentrate list to list.
- Example #2. What is the output of the following program?
Output:
[42, 42, 42]
This is because += operator can be used to concentrate tuple to list.
- Example #3. What is the output of the following program?
Output:
Error
This is because we can’t append nothing to a list, append must have a parameter.
- Example #4. What is the output of the following program?
Output:
[42, 42, None]
This is because None is not nothing, it is actually a type of data.
- Example #5. What is the output of the following program?
Output:
Error
This is because we can’t expend the list with a single value.
- Example #6. What is the output of the following program?
Output:
[42, 42, '4', '2']
This can be hard to answer because people forget the string is actually a list of chars in this case. If we want to add ‘42’ directly in the end, we have to use append to treat that as a whole, which is,
A = [42, 42]
B = '42'
A.append(B)
print(A)
- Example #7. What is the output of the following program?
Output:
Error
Even if we use the parentheses for B, this still means an integer instead of a tuple. So it can not be extended to a given list.
- Example #8. What is the output of the following program?
Output:
[42, 42, 42]
This program is equivalent to,
A = [42, 42]
B = [42]
A.extend(B)
print(A)
- Example #9. What is the output of the following program?
Output:
[42, 42, 42]
The list can be extended by a set. Note that the element of a set can not be a list (tuple is okay).
- Example #10. What is the output of the following program?
Output:
[42, 42, 42, 42]
(4) List Slicing
Note that we can use [::-1] to reverse a list.
- Example #1. What is the output of the following program?
Output:
[]
By default, the step of slicing will be set to positive 1.
- Example #2. What is the output of the following program?
Output:
[6, 5, 4]
We can slice out of the range of the list.
- Example #3. What is the output of the following program?
Output:
Error
(5) List Copy
Because the list is mutable, so we can not assign a list to another with an assigning statement. We have to use .copy()
method for assigning.
- Example #1. What is the output of the following program?
Output:
[42, 42]
- Example #2. What is the output of the following program?
Output:
[1, 42]
(6) List Element Types
The element in a list must be an object. For example, all functions can be elements in the list while some of the reserved keywords can not be put into the list.
- Example #1. What is the output of the following program?
Output:
True
- Example #2. What is the output of the following program?
Output:
Error
(7) Other List Methods
- Example #1. What is the output of the following program?
Output:
[1, 2, 3, 4]
We just write the function in the second line, but we didn’t apply it to the list. Therefore, the result won’t change. If we want to clear the list, we have to type the parenthesis.
nums = [1, 2, 3, 4]
nums.clear()
nums
Note that the clear method takes no arguments, or it will return us an error.
- Example #2. What is the output of the following program?
Output:
[2, 3, 2, 2]
This will count the occurring times of all elements in the list.
- Example #3. What is the output of the following program?
Output:
5
This is the same as,
print(nums[3:6].index(2) + len(nums[0:3]))
- Example #4. What is the output of the following program?
Output:
['i', 1, 2, 4, 1, 5, 2, 2, 3, 4]
The index we provide for the insert method can be negative, and it can also be out of the range of the list.
- Example #5. What is the output of the following program?
Output:
2
[1, 4]
We can pop any element by its index. This index can be negative but can not be out of range.
- Example #6. What is the output of the following program?
Output:
Error
Can not pop from an empty set.
- Example #7. What is the output of the following program?
Output:
None
[1, 2, 4, 1, 5, 2, 2, 4]
Note that remove is to remove the first value of the argument in the list, while pop is to pop the value locates in the argument index. It is also important to know that the remove method returns no value.
(8) Tuple Methods
There are only two methods for a tuple: .count()
and .index()
. The tuples can also do slicing.
- Example #1. What is the output of the following program?
Output:
[2, 3, 1, 2, 1]
This is quite similar to a list.
- Example #2. What is the output of the following program?
Output:
Error
We can not assign by the index of a tuple. If we must change the value of a tuple, we can use the following code,
A = (42, 42)
B = list(A)
B[0] = 1
print(A)
print(tuple(B))
this will give us,
(42, 42)
(1, 42)
- Example #3. What is the output of the following program?
Output:
1
2. List Comprehension
(1) List Comprehension
The list comprehension is a cool way to generate a list. It has the form of,
(return) [<fun> for <item> in <sequence> (if <condition>)]
The function in this could be any function, including the in-built functions, the functions from packages, or the functions defined by us.
- Example #1. What is the output of the following program?
Output:
H
l
l
W
r
l
d
!
[None, None, None, None, None, None, None, None, None]
- Example #2. What is the output of the following program?
Output:
[0, 1, 4]
This is the same as,
x = [i**2 for i in range(3)]
print(x)
(2) The map Object
The general form of a map object can be calculate through the map function. The general form of the map function is,
map(func, *iterables)
The func function can be any function, including the in-built functions, the functions from packages, or the functions defined by us. The map object is to take each of the iterables as the an argument of this function.
- Example. What is the output of the following program?
Output:
0
1
2
3
4
[None, None, None, None, None]
(3) lambda Functions
lambda
functions in Python are anonymous functions. lambda
functions are also called in-line or throw-away functions. For example, if we write,
lambda n: n*n
It is equivalent to write (can not run the following code, because we will have namespace problem),
def fun(n):
def square(n):
return n * n
return square
So that if we have,
tuple(map(lambda n: n*n, range(11)))
this is equivalent to,
def square(n):
return n * n
tuple(map(square, range(11)))
- Example. What is the output of the following program?
Output:
[7, 9, 11, 13, 5]
(4) filter Function
The filter has a similar constructure of the map function, which is,
filter(function or None, iterable)
It is different from the map because it doesn’t contain any return value from the function. In fact, the filter uses the return value of the function as a boolean value and use this value to judge whether or not putting the iterables into the filter. If the return value from the function is false, then we just ignore its argument and the filter will not contain the particular argument value of this function.
- Example. What is the output of the following program?
Output:
0
1
2
3
4
[]
(5) reduce Function
The reduce is not a in-built function, so we have to import it before we use it.
from functools import reduce
For everytime of the calculation, the lambda (or the other given function) will take in two values, and it is going to return one value. In the next iteration, it will treat this result as the first argument and then put in another new iterable as the second argument.
- Example #1. What is the output of the following program?
Output:
15
- Example #2. What is the output of the following program?
Output:
little
3. General Questions
- Example #1. What is the output of the following program?
Output:
Error
In a lambda function, statements like return
, pass
, assert
, or raise
will raise a SyntaxError
exception.
- Example #2. What is the output of the following program?
Output:
([1, 2, 0], [4, 5, 6])
We can change the mutable element in an immutable one.