Advanced Python 5 | Set, Dictionary, and Counter
Advanced Python 5 | Set, Dictionary, and Counter
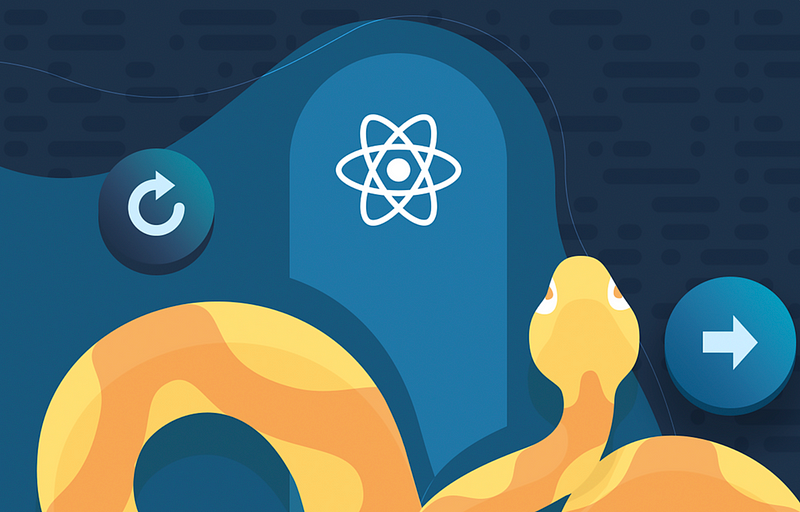
- Set Methods
(1) Three Features of the Sets
There are 3 important features of sets. Sets are collections that are:
- unique elements
- mutable, but the elements in a set must be immutable (hashable)
- unordered
By the previous code, we can also list all the methods of the set.
[method for method in dir(set) if not method.startswith("__")]
then,
['add',
'clear',
'copy',
'difference',
'difference_update',
'discard',
'intersection',
'intersection_update',
'isdisjoint',
'issubset',
'issuperset',
'pop',
'remove',
'symmetric_difference',
'symmetric_difference_update',
'union',
'update']
(2) Set Methods
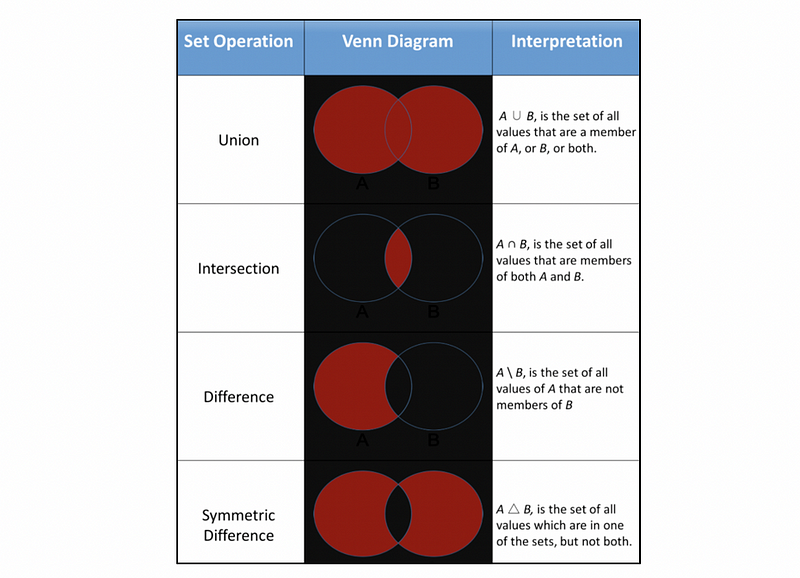
Suppose we have two sets, old and new, then,
- union
new.union(old)
- intersection
new.intersection(old) # or new & old
- difference
new.difference(old) # or new - old
- symmetric difference
new.symmetric_difference(old) # new ^ old
- add an element to the set (in-place function) (no change if already exists)
new.add('a')
- copy a set (because set is mutable)
New = new.copy()
- update a set with its union with another set (in-place) (which is also be considered as union_update but they just define this name like that)
new.update(old) # the same of new = new.union(old)
- update a set with its intersection with another set (in-place)
new.intersection_update(old)
- update a set with its difference with another set (in-place)
new.difference_update(old)
- update a set with its symmetric difference with another set (in-place)
new.symmetric_difference_update(old)
- discard an element in this set (in-place) (when this element does not exist, it will in-place the original set)
new.discard('a')
- randomly pop an element form the set (in-place, return the poped element)
new.pop()
- check whether two set not have a same element
new.isdisjoint(old)
- check whether a set is the subset of another set
new.issubset(old)
- check whether a set is the superset of another set
new.issuperset(old)
- clear a set (in-place)
new.clear()
- Example #1. What is the output of the following program?
Output:
None
This is because update is an in-place method.
- Example #2. What is the output of the following program?
Output:
{1, 'PYnative', ('abc', 'xyz')}
This is because the hash for True and the hash value for 1 are the same.
- Example #3. What is the output of the following program?
Output:
Error
This is because the list is not hashable.
- Example #4. What is the output of the following program?
Output:
{'Green', 'Orange', 'Black', 'Blue', 'Red', 'Yellow'}
Note that the argument for method union
, update
, differnce
, differnce_update
, intersection
, intersection_update
, symmetric_difference
, symmetric_difference_update
, isdisjoint
, issubset
, and issuperset
can be set, list, tuple, or any kinds of iterable data type.
(3) Set application
- Eliminate Duplicated Elements
By changing a list to a set, we can eliminate all the duplicated elements in that list.
set(myList)
- Membership Testing
It will be faster if we use set for membership test compare with a list.
anitem in set(myList)
2. Dictionary Methods
(1) Dictionary Methods In A Nutshell
The feature of the dictionary is that the dictory itself is mutable but the keys in the dictionary must be immutable.
[method for method in dir(dict) if not method.startswith('__')]
then,
['clear',
'copy',
'fromkeys',
'get',
'items',
'keys',
'pop',
'popitem',
'setdefault',
'update',
'values']
(2) Dictionary Methods (Suppose we have dictionary cart)
- return the keywords of a dictionary (the return type is a
dict_keys
type and this can be iterated)
cart.keys()
- return the values of a dictionary (the return type is a
dict_values
type and this can be iterated)
cart.values()
- return list of tuples of keywords and keywords of a dictionary (the return type is a
dict_items
type and this can be iterated)
cart.items()
- Never use keyword index: keyword index is definitely not a good idea because if you haven’t got a key inside a dictionary then it will raise an error. We can use this to assign the value of a keyword and this is a good to do.
cart['apple'] # avoid using things like this to get a value
- get the value by keyword index with one argument keyword. This is a much better way because it will return None if there this keyword doesn’t exist.
cart.get('Apples')
- get the value by keyword index, if this keyword doesn’t exist, add this keyword to the dictionary and assign the value of this keyword as the second argument. This is always being used to initialize a dictionary.
cart['Apples'] = cart.get('Apples', 0)
Or we can use this to get any value by index.
cart.get('Limes', "Not on in the shopping cart")
- pop an item by keyword, this will return the poped value (in-place). We can not pop a keyword that doesn’t exist in this dictionary.
cart.pop()
- randomly pop an item, this will return the poped item in a tuple (in-place)
cart.popitem('Apples')
- add a new item to the given dictionary (in-place)
cart.update({'Banana': 5})
or
cart.update(Banana=5)
or
cart.update([(Banana,5)])
- change the value of an existing keyword in the dictionary
cart.update({'Apples': 5})
- set a default None value to a not existing keyword, and return None if the keyword doesn’t exist, while return the value of this keyword if the keyword exists (if the keyword exists, it won’t change anything) (in-place)
cart.setdefault('Apples')
- setup a dictionary from an iteration of keys, the values will be initialized to None
dict.fromkeys(myList)
We can also assign the same value for all the keywords.
dict.fromkeys(myList, myValues)
- copy and assign a dictionary
new_cart = cart.copy()
- clear a dictionary
cart.clear()
- remove an item from the dictionary (not using method, if we want to use the method, use pop, and we haven’t got remove here)
del cart['Apples']
- Example #1. What is the output of the following program?
Output:
{'a': [1, 2], 'u': [1, 2], 'o': [1, 2], 'e': [1, 2], 'i': [1, 2]}
This is because list is mutable.
- Example #2. What is the output of the following program?
Output:
{'Apples': 3, 'Oranges': 3}
{'Oranges': 3, 'Apples': 3}
True
This is because Python 3.6 keeps insertion order in dictionaries. But it doesn’t contain a real order, so the dictionaries with different insertion order can still be equal.
- Example #3. What is the output of the following program?
Output:
Error
- Example #4. What is the output of the following program?
Output:
None
Note that we have get in this case.
(3) Tricky Staff with List Comprehension
- Sort the insertion order of a dictionary by value
dict(sorted(d.items(), key=lambda x: x[1]))
- Find keys in a dictionary that match a value
[k for k, v in myDictionary.items() if v == target_value]
- randomly pick an item
import random
random.choice(list(d.items()))
3. Counter
Counter is a special type of dictionary that count the number of each unique element in a list. This is not a built-in function so we have to import it at the front.
from collections import Counter
For example, if we run
Counter("abracadabra")
then,
Counter({'a': 5, 'b': 2, 'r': 2, 'c': 1, 'd': 1})
This is commonly used in the settings of an NLP problem.
4. General Questions
- Example #1. What is the output of the following program?
Output:
{1: 2}
functions are allowed for the keyword of the dictionary, things are not immutable(if/not/else/for/dict/list/etc…) are not allowed. For several keywords with the same context, we can only get the last one by indexing.
- Example #2. What is the output of the following program?
Output:
Error
Can not update an empty set.
- Example #3. What is the output of the following program?
Output:
{1: 1, 2: 2, 3: 3}