Advanced Python 7 | OS/SYS Module, File I/O, and pathlib
Advanced Python 7 | OS/SYS Module, File I/O, and pathlib Module
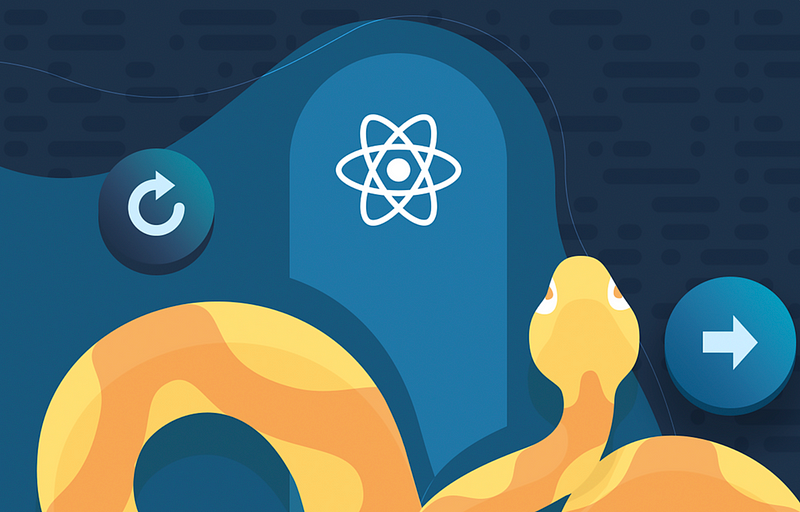
- OS Module
Before we use the OS Module, first of all, we have to use import this module,
import os
- get the os name (usually ‘POSIX’)
os.name
- get the current path
os.getcwd()
- make a new folder
os.mkdir('newDir')
- rename a folder
os.rename('newDir','newDir2')
- delete a folder
os.rmdir('newDir2')
2. SYS Module
Before we use the SYS Module, first of all, we have to use import this module,
import sys
- see the environment paths, return a list of paths
sys.path
- see the platform of the system
sys.platform
- see the path of the python interpreter
sys.executable
- get a tuple of all the build-in modules
sys.builtin_module_names
we will have,
('_abc',
'_ast',
'_codecs',
'_collections',
'_functools',
'_imp',
'_io',
'_locale',
'_operator',
'_signal',
'_sre',
'_stat',
'_string',
'_symtable',
'_thread',
'_tracemalloc',
'_warnings',
'_weakref',
'atexit',
'builtins',
'errno',
'faulthandler',
'gc',
'itertools',
'marshal',
'posix',
'pwd',
'sys',
'time',
'xxsubtype',
'zipimport')
- get a set of all the modules (i.e. pandas, NumPy, etc…)
sys.modules
- input by standard input
sys.stdin.readline()
Actually, we can rebuild the inbuilt input() function with stdin and stdout.
To test this, we can input anything we want, for example, we can input,
12
then,
Please input: 12
the output is: 12
Please input: 12
the output is: 12
- print by standard output
sys.stdout.write()
We can also rebuild the inbuilt print() function with stdout.
Run this code, we will then have,
hello, world!
hello, world!
- get the recursion limit
Recall what we have talked about of the recursion. In python, there is a recursion limit and we can get this limit by,
sys.getrecursionlimit()
- set the recursion limit
We can also reset this limit, i.e. we can change this to 100.
sys.setrecursionlimit(100)
- get the reference count of an object
sys.getrefcount(object)
Click this link to get more information about the reference count.
- get the bytes of an object
sys.getsizeof(object)
3. File I/O
- Get the hexadecimal of a file in macOS terminal (show 96 bytes)
$ hexdump -n 96 one_fish.txt
$ hexdump -n 96 i_am_not_text.jpg
- Get the hexadecimal of a file in Linus CLI (show 96 bytes)
$ xxd -l 96 one_fish.txt
$ xxd -l 96 i_am_not_text.jpg
- open a file and then close a file (Remember to CLOSE it)
f = open("students.txt")
f.close()
Note that two file objects will not be the same before and after closing.
- open a file by
with
statement (automatically closed)
with open("students.txt") as f:
print(f)
This will give us the information about this file object.
- read a line of a file object, return a string, with \n at the end
f.readline()
- read all of a file object, return a string
f.read()
- read all of a file object, return a list with each element is a line
f.readlines()
- write to files
First of all, the file should be open by ‘w’ mode
f = open(filename, 'w') # or we can use the with statement
Then write ‘hi’ to this file,
f.write('hi')
Remember to close the file,
f.close()
4. pathlib Module
Before we use the Path Module, first of all, we have to use import this module,
from pathlib import Path
- read all of a file object by Path
Path("students.txt").read_text()
- define a path with Path object
path = Path('../data/')
- get the current path
pwd = Path.cwd()
- get the home path
home = Path.home()
Note that the path object is not a string, but a pathlib.PosixPath
. It is kind of a special string.
- create a new path by a path object
new = Path.cwd() / 'data'
this will add /data after our current path.
- write files by Path
Path("students.txt").write_text('hi')
- return the whole path by filename
Path("students.txt").resolve()
- return the only the suffix of a file
Path("students.txt").resolve().suffix
- return the filename without the suffix
Path("students.txt").resolve().stem
- return the filename with the suffix
Path("students.txt").resolve().name()
- return the path of the folder where the file located
Path("students.txt").resolve().parent()
- return an iterator of all the file paths and file paths in the folders in the given path (recursion)
path = Path.cwd()
path.rglob('*.*')
- return an iterator of all the .txt file paths and .txt file paths in the folders in the given path (recursion)
path = Path.cwd()
path.rglob('*.txt')
- return an iterator of all the file paths in the current path
path = Path.cwd()
path.glob('*.*')
- return an iterator of all the .txt file paths in the current path
path = Path.cwd()
path.glob('*.txt')
- return an iterator of all the file paths in the current path and the level one folders
path = Path.cwd()
path.glob('*/*.*')
- return an iterator of all the file paths in the current path and recurse all the folders inside
path = Path.cwd()
path.glob('**/*.*')
5. Practices
(1) Example #1. What will be the content of the hello.txt?
Answer:
yes
nono
yes
(2) Example #2. What will be the output of this program?
Answer:
<_io.TextIOWrapper name='exec.txt' mode='a' encoding='UTF-8'>
A closed file is still a file object.
(3) Example #3. What will be the output of this program?
Answer:
False
Even if two file objects are properly closed, they are not the same.
(4) Example #4. What will be the output of this program?
Answer:
False
Same.
(5) Example #5. What will be the output of this program?
Answer:
True
This is a simple assigning statement.