Play With the Command Lines
Play With the Command Lines
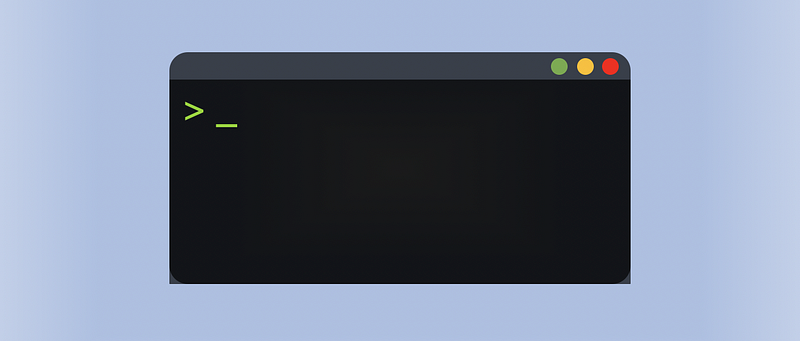
- ssh connection (with no certificate, i.e. .pem, and the password is always required)
ssh <username>@<domain/IPaddress> -p <port>
- ssh connection (with RSA certificate sshkey.private)
ssh -i sshkey.private <username>@<domain/IPaddress> -p <port>
- log out the ssh connection
exit
- list the contents in the current directory
ls
- list all the contents (include the hidden files) in the current directory
ls -a
- list all the contents and the information of the files in the current directory
ls -l
- display all the filetypes in the current directory
file *
- open a file with nano
nano <filename>
- open a file with vim
vi <filename>
- open another file with vim (without closing it)
:e <path/filename>
- open a file with emacs
emacs <filename>
- open a file named - (or begin with - )
nano ./-
- open a file has spaces in its name
nano spaces\ in\ this\ filename
- output the content of a file
cat <filename>
- send back a message
echo <msg>
- go into a folder
cd <foldername>
- go back to the parent folder
cd ..
- go back to the last path
cd -
- reset the terminal format
reset
- change the mode of a file
chmod <ModeCode> <filename>
Common mode codes are:
777 # everyone can rwx
755 # the owner can rwx, other can only rw
644 # the owner can only rw, other can only r
655 # the owner can only rw, other can only rx
400 # only the owner can read
- output the first 1–3 letters of the stdout (Hel)
echo Hello, World! | cut -c 1-3
- output the content before ‘,’ in the stdout (Hello)
echo Hello, World! | cut -d ',' -f 1
- output the content before ‘ ’ in the stdout (Hello,)
echo Hello, World! | cut -d ' ' -f 1
- find the file by its name (* can be used)
find . -name <filename>
- find file by its type (d/f/*.txt/etc..)
find . -type <filetype>
- find the file by its mode (0777/0400/etc..)
find . -type f -perm <mode>
- find the file by its size
find . -size <filesize>
- find and change mode
find . -type f -perm <mode1> -print -exec chmod <mode2> {} \;
- find human-readable files
find . -exec file {} + | grep ASCII
- find the file with multi conditions
find . \
-size 1033c \
-not -executable \
-exec file {} + | grep ASCII
- find a specific file from the root (with a specific user and a specific group) without the root permission
find / \
-user bandit7 \
-group bandit6 \
-size 33c
- show content of a file in one page (controlled by arrow keys)
cat <filename> | less
or
less <filename>
- show content of a file in one page (controlled by entering keys)
cat <filename> | more
or
more <filename>
- interrupt the more/less command and then go to the vim editor (when there’s a more/less shown)
v
- print the line in a file with a specific word
cat <filename> | grep <word>
- print the count (number) of the matching lines in a file
cat <filename> | grep -c <word>
- print the index and the matching line in a file
cat <filename> | grep -n <word>
- filter all lines that match the character
cat <filename> | grep -i <char>
- filter all lines that do not contain a character
cat <filename> | grep -v <char>
- sort all lines in a specific file
cat <filename> | sort
- reversely sort all lines in a specific file
cat <filename> | sort -r
- find the unique lines in a file (because the unique command only treats the continuous repeated lines as not unique, so we have to sort the lines before we use this command). -u means to print only the unique lines but the unique command without any options means to display all the non-unique lines (which can be weird to some extent).
cat <filename> | sort | unique -u
- count the time that each line repeats
cat <filename> | sort | unique -c
- print the possible human-readable parts in a data type file
cat <filename> | strings
- encode a file by base64 encoding
cat <filename> | base64
- decode a file by base64 encoding
cat <filename> | base64 -d
- encode/decode a file by ROT13 encoding
cat <filename> | tr "A-Za-z" "N-ZA-Mn-za-m"
- make new directory
mkdir <path/dirname>
- copy files to a new directory
cp <file> <directorypath>
- rename a file
mv <oldfilename> <newfilename>
- change a plain text file to a hex dump ASCII file
xxd <filename>
- change a hex dump ASCII file back to the origin file
xxd -r <filename>
- unzip a .gzip file
gzip -d <filename>
- unzip a .bz2 file
bzip2 -d <filename>
- unzip a .tar file
tar xvf <filename>
- delete any files/folders
rm -rf <file/folder>
- telnet is a previous version of network connection similar to ssh, this is an instance of the Star War visualization in terminal
telnet towel.blinkenlights.nl
- we can also use telnet to connect to our localhost port 80 (because this is always open)
telnet -r localhost 80
we have to type in the password of our PC in order to connect to this port.
- to connect to any given IP and given port that supporting telnet connection, we can use
telnet -r <IPaddress> <port>
- connect to the localhost 80 port by SSL (this command will set our terminal as a client)
openssl s_client -connect localhost:80
- scan all the ports on the localhost
nmap -p - localhost
- scan the ports from 31000 to 32000 on the localhost, return only the ports
nmap -p 31000-32000 localhost | grep -E ^31 | cut -c1-5
- find out the all the ports that uses the ssl
nmap --script ssl* -p - localhost
- find the difference between two files (the content in file1 will be output first)
diff <file1> <file2>
- when bash is configured in .bashrc to log you out when you are connected with ssh, we can have three ways to go across this
(1) Run the code directly when doing ssh connecting (i.e. cat readme)
ssh <username>@<domain/IPaddress> -p <port> cat readme
(2) Using a pseudo-terminal (use sh/dash instead of bash)
ssh <username>@<domain/IPaddress> -p <port> -r /bin/sh
(3) Ignore the shell (.bashrc) on the server (use sh/dash/bash/etc. on your PC instead of bash)
ssh <username>@<domain/IPaddress> -p <port> /bin/sh
- get the current user
whoami
- get the current user and the current group
id
- run the command with another user with a setuid file
<path/setuidfilename> <command>
- listening to a given port on the localhost by netcat (set as a server)
nc -l <port>
- connect to a given port on the localhost by netcat (set as a client)
nc <domain/IPaddress> <port>
- listening to a given port on the localhost by netcat (set as a server) decoding the information by base64
nc -l <port> | base64 -D
- connect to a given port on the localhost by netcat (set as a client) encoding the information by base64
echo <msg> | base64 | nc <domain/IPaddress> <port>
- output the status of the last command (0 if successful, 1 if failed)
echo $?
- redirect the stdout to a file named logout (1>)
ping 8.8.8.8 1> logout
- redirect the stderror to a file named error (2>)
asdasd 2> error
- ignore all the stdout (redirect the stdout to /dev/null)
ping 8.8.8.8 1> /dev/null
- ignore all the stderror (redirect the stderror to /dev/null)
asdasd 2> /dev/null
- redirect the stderror to stdin
echo test 2>&1
- redirect the stdin to stderror
echo test 1>&2
- ignore all the stdout and stderror (redirect the stdout and stderror to /dev/null)
echo test &> /dev/null
- edit the corntab time table to set up a time for a program
corntab -e
- set the time when a program will execute (after corntab -e)
***** <username> <command>
Note that the formatting of the time in this should follow this link.
- make an SH file (named with xxx.sh)
#!/bin/bash
<command #1>
<command #2>
<command #...>
- set values to variables in the shell (varname: value)
<varname>=<value>
Note that there should be no spaces in this command.
- set stdouts to variables in the shell (varname: stdout)
<varname>=$(<command>)
- get the hash value of a string by md5
echo <string> | md5sum
we can also eliminate the dash sign by the cut command
echo <string> | md5sum | cut -d ' ' -f 1
- for loop by a sequence 1 2 3 4 5 with bash
- for loop by a sequence 1 2 3 4 5 (a brief shortcut) with bash
- for loop by a sequence with step 1 3 5(a brief shortcut) with bash
- for loop by a sequence 1 2 3 4 5 (a c-like way) with bash
- for infinite loop with bash
- exit the session in the current terminal
exit 1
- read from stdin to assign a variable
read VAR
- test if a file exists
test -a <filename>
- test if a file is readable
test -t <filename>
- test if two strings are equal
test <str1> == <str2>
- test if two strings are not equal
test <str1> != <str2>
- test if two numbers are equal
test <num1> -eq <num2>
- test if two numbers are not equal
test <num1> -ne <num2>
- test if the first number is greater than the second one
test <num1> -gt <num2>
- test if the first number is greater than or equal to the second one
test <num1> -ge <num2>
- test if the first number is less than the second one
test <num1> -lt <num2>
- test if the first number is less than or equal to the second one
test <num1> -le <num2>
- if…then…else…fi condition statement with bash (An Example)
- rewrite a file with a line of string
echo <string> > <path/filename>
- append a string to the end of a file
echo <string> >> <path/filename>
- rewrite a file with another file
cat <filename> > <path/filename>
- show all the shells we can use
cat /etc/shells
- show which specific shell each user is using
cat /etc/passwd
- let vim show the present shell
:set shell?
- reset the shell through vim (i.e. to bash)
:set shell=/bin/bash
- run commands through vim
:! <command>
- clone a git repo by ssh
git clone <sshAddress>
- see the short hash value of the last commit
git reset --hard
- revert back to a previous version by given short hash value
git revert <ShortHash>
- check the status of the present git
git status
- pull the stuff in the git status to the present version
git pull
- show the log of every git commit
git log -p
- show the local git branches
git branch
- show the remote git branches
git branch -r
- switch git branches
git checkout <banchname>