High-Performance Computer Architecture 1 | The Risc Assembly Refresher
High-Performance Computer Architecture 1 | The Risc Assembly Refresher
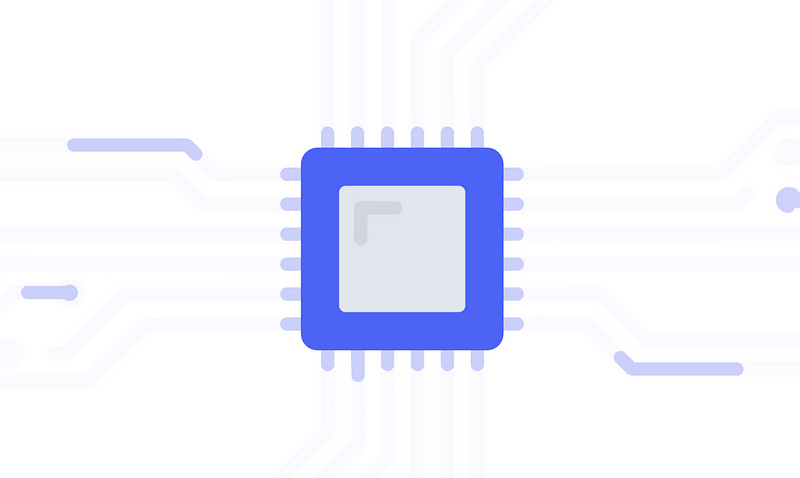
A reduced instruction set computer, or RISC, is a computer with a small, highly optimized set of instructions. Microprocessor without Interlocked Pipelined Stages (MIPS) is a special kind of RISC architecture. The instruction set of the MIPS64 can be found here.
- Basic Calculation
- Add with overflow the values in the register R2 and R3 and put the result in register R1,
ADD R1, R2, R3
Or,
ADD R1 = R2 + R3
- Subtract with overflow the values in registers R1 and R5 and put the result in register R4,
SUB R1, R4, R5
- Multiply the values in registers R8 and R9 and put the result in register R5,
MUL R5, R8, R9
- Divide the value in register R3 by the value in register R4, store the result in register R1,
DIV R1, R3, R4
- Exclusive-Or the bit values in registers R7 and R8 and put the result in register R6,
XOR R6, R7, R8
Note the XOR calculation follows the rules of,
0 0 1 1
XOR 0 XOR 1 XOR 0 XOR 0
------- ------ ------- -------
0 1 1 0
2. Bouncing Operations
- Compare the value in registers R1 and R2. If they are equal, then jump to the instruction labeled “Label”,
BEQ R1, R2, Label
- Compare the values in registers R1 and R3. If they are not equal, jump to the instruction labeled “Label”,
BNE R1, R3, Label
3. Memory Operations
- Get the value stored in register R6, add 0 to the value. Use this sum as an address, then get the value at this address and store it in register R3,
LOAD R3 = 0(R6)
- Get the value in the R2 register, add 34 to that value, then use the result of the addition as a memory address to write to that address and put the value of register F6 into that memory location,
LD F6, 34(R2)
- Get the value stored in register R7, add 0 to this value. Use the sum as an address, and store the value in R4 at this address,
STORE R4 -> 0(R7)
- Get the value stored in R2, add 0 to it. This is the address to be accessed. Get the value stored in register R1 and store it in the accessed address,
SW R1 -> 0(R2)
Or,
SW R1, 0(R2)
- Get the value stored in R2, add 0 to it. This is the address to be accessed. Get the value at the accessed address and store it in the register R2,
LW R2 <- 0(R2)
Or,
LW R2, 0(R2)