High-Performance Computer Architecture 2 | The C++ Refresher
High-Performance Computer Architecture 2 | The C++ Refresher
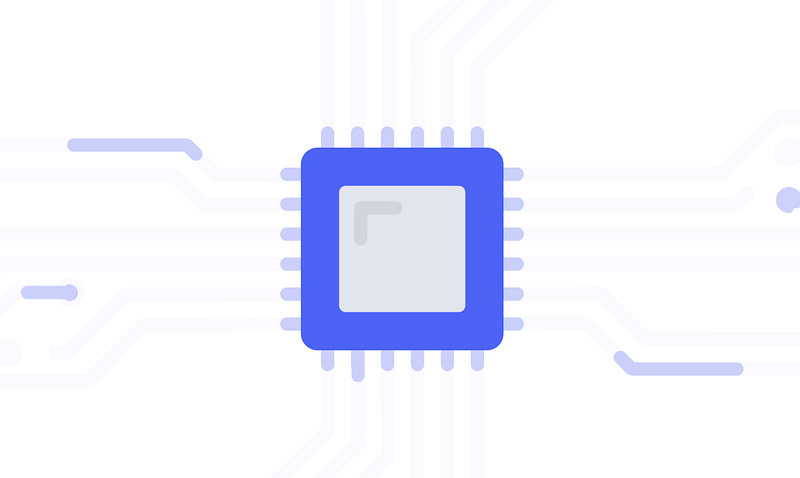
- The basic C++ Program
// A basic C++ Program
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!";
}
- Line 1: the double slashes
//
are used to denote this line as a comment. - Line 3: the
#
symbol means this line is a preprocessor directive. The preprocessors are the directives, which give instructions to the compiler to preprocess the information before the actual compilation starts. Preprocessor directives are not C++ statements, so they do not end in a semicolon;
. This command requests the standard IO header fileiostream
be included. - Line 4: this line means that we are using the standard library, which means that
std::cout
is equivalent tocout
. - Line 6: this line is the function declaration, which gives the name of the function as
main
.int
means this function returns an integer and because there are no parameters given in the parentheses()
, the function accepts no inputs. - Line 6 and 8: the curly brackets
{}
enclose all the code for the function. - Line 7:
cout << "Hello World!"
means to produce the outputHello World!
2. Variable Types in C++
int
: signed integer, 4 bytes = 32 bitsunsigned int
: unsigned integer, 4 bytes = 32 bitssigned int
: signed integer, 4 bytes = 32 bitsshort
: signed short integer, 2 bytes = 16 bitsunsigned short
: unsigned integer, 2 bytes = 16 bitssigned short
: signed integer, 2 bytes = 16 bitslong
: signed ling integer, 8 bytes = 64 bitsunsigned long
: unsigned integer, 8 bytes = 64 bitssigned long
: signed integer, 8 bytes = 64 bitschar
: character, 1 byte = 8 bitsunsigned char
: unsigned character, 1 bytes = 8 bitssigned char
: signed character, 1 bytes = 8 bitsfloat
: floating point number, 4 bytes = 32 bitsdouble
: double-precision floating point number, 8 bytes = 64 bitsbool
: boolean value, 1 byte = 8 bits*
: integer pointer, 8 bytes = 64 bits[]
: array,sizeof(datatype) * amount
3. Declaring Constants
#define
defines a macro, which allows the programmer to give a name to a constant value before the program is compiled. Because the macro is different from a variable, so we can not change the value of this macro.static
defines a global variable, which allows a variable to be visible for all the functions. We can change the value of this variable, so this variable is not constant. Actually, we don’t have to add the static in most cases to declare a global variable.const
defines a constant. It implies that the current value of the variable can not be reassigned. Note that a constant variable doesn’t mean that it is a global variable.
4. Increment and Decrement
We have 4 ways to increase an int variable x
by 1,
++x;
x++;
x+=1;
x=x+1;
Note that the difference between ++x;
and x++;
appears when we assign the value to another variable. For example, if we assign this value to a variable y
,
y = ++x;
means,
y = x + 1;
x = x + 1;
And,
y = x++;
means,
y = x;
x = x + 1;
5. If-Else Statement
The structure of the if-else statement in C++ is similar to C,
if (x>0) {
} else if (x<0) {
} else {
}
6. Looping Statement
- Infinite
while
loop
while (1) {
}
- Infinite
for
loop
for ( ; ; ) {
}
- Infinite
do while
loop
do {
} while (1);
- Finite
while
loop
int n = 10;
while (n>0) {
n--;
}
- Finite
for
loop
for (int n = 10; n > 0; n--) {
}
- Finite
do while
loop
int n = 10;
do {
n--;
} while (n>0);
7. Switch Statement
switch (x) {
case 1:
/* something */
case 2:
/* something */
default:
/* something */
}
8. Functions
#include <iostream>
using namespace std;
void hello(void);
int main(void) {
hello();
return 0;
}
void hello(void) {
cout << "Hello World!"
}
- Line 4: this line is used to declare the function
- Line 7: this line is used to call the function
- Line 11–13: these lines are the function.
9. Array
int a[2] = {1, 2};
cout << a;
cout << a + 1;
cout << &a[0];
cout << &a[1];
cout << a[0];
cout << a[1];
cout << *a;
cout << *(a+1);
- Line 1: define and initialize an array
- Line 3, Line 5: print the address of the first value
- Line 4, Line 6: print the address of the second value
- Line 7, Line 9: print the first value
- Line 8, Line 10: print the second value
10. Class
#include <iostream>
using namespace std;
class Rectangle {
int width, height;
public:
void set_values(int, int);
int area() {
return width * height;
}
};
void Rectangle::set_values(int x, int y) {
width = x;
height = y;
}
int main() {
Rectangle rect;
rect.set_values(3, 4);
cout << rect.area();
return 0;
}
- Line 4: defining the
Rectangle
class - Line 5: these variables are accessible only by code in the
Rectangle
class - Line 6: means the following codes can be called by code both inside and outside the
Rectangle
class - Line 11: remember to add a
;
after a class definition - Line 13: define the public member
set_values
- Line 19: declaring a variable called
rect
to be an instance of theRectangle
class - Line 20: setting the
width
andheight
variables - Line 21: using the rectangle member
area()
to calculate the area of therect
.