Java Programming 1 | Built-in Data Types, I/O, Computing, Java’s Math Library, and Type Conversion
Java Programming 1 | Built-in Data Types, I/O, Computing, Java’s Math Library, and Type Conversion
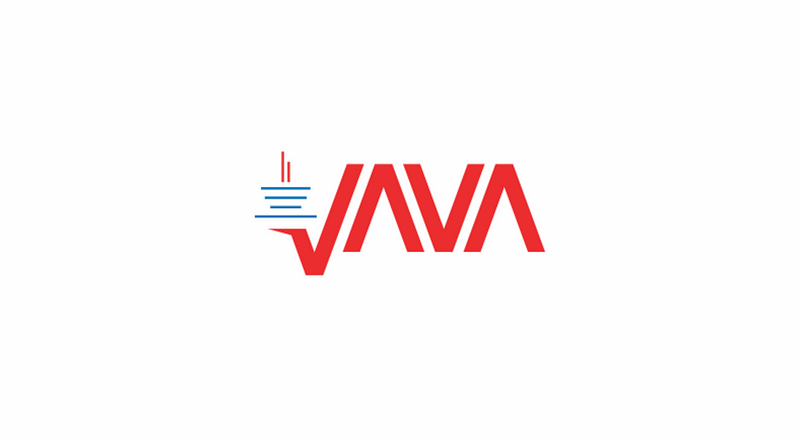
- Setting Up Java Environment
Before we started, we have to install java programming environments on our computers. See this link to install the latest IntelliJ onto our computer. Then, test the following code in your IntelliJ.
To run this code, we can press control (^)+ shift (⇧) + letter R, then IntelliJ will compile and execute this code for us. Another way is to compile and execute this code from the command line. We can press Command (⌘) + letter B to compile the file and then press Command (⌘) + letter E to execute.
First, we go into our path, it is going to show in our IntelliJ IDE. Then it would be a good idea if we type in CLI,
$ java HelloWorld.java
then we can have,
Hello, World
This means that the environment of Java is successfully set up on our computer. Congratulations!
2. Our First Java Programme
Now, let’s look back at our HelloWorld.java program. It has:
- a program name
- a main() method
- body of the main method
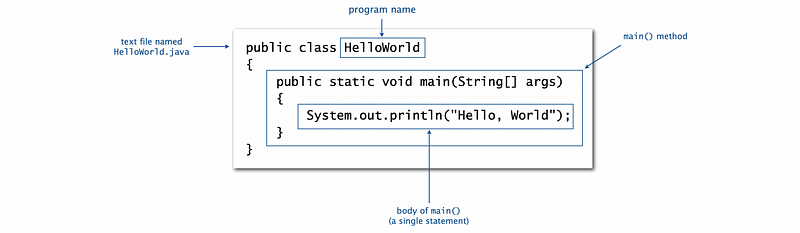
3. Built-in Data Types
(1) What Is a Data Type?
A data type is a set of values and a set of operations on those values.
(2) Built-in Data Types
There are basically five built-in data types in java, which are:
- char
- String
- int
- double
- boolean
And you can remember them through the following graph,
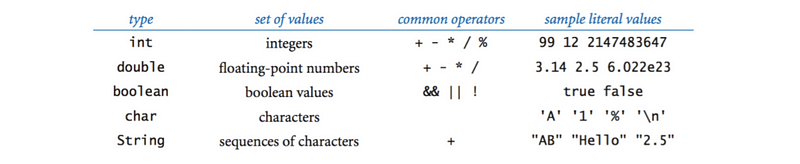
(3) Basic Definitions in Java (Or Other Programming Language)
- Variable
A variable is a name that refers to a value.
- Literal
A literal is a programming-language representation of a value.
- Declaration Statement
A declaration statement associates a variable with a type.
- Assignment Statement
An assignment statement associates a value with a variable.
Note that we can combine the declaration statement and the assignment statement.

- Trace
A trace is a table of variable values after each statement.
4. Input and Output
If we want to read the data from the command line, we have to call the system method to convert the strings that we typed in into numbers,
Integer.parseInt()
We can also call the system method,
System.out.println()
for us to print some variables. Let’s do a practice to find out what will the following program do if we type in 1234 99 as our argument,
This program helps us to swap two numbers. Suppose you try it in IntelliJ, our result is:
99
1234
5. Data Type for Computing
(1) String
For stings, we can concatenate two strings together by operator +. For example, with the following code as,
is going to give us the result of,
121312141213121
(2) int
For integrate data type, we can do math calculations through different operators: +
(add), -
(subtract), *
(multiply), /
(divide), and %
(remainder). For example, if we give the argument 1234 99, then
the code above will give us the result,
1234 + 99 = 1333
1234 - 99 = 1135
1234 * 99 = 122166
1234 / 99 = 12
1234 % 99 = 46
(3) boolean
For integrate data type, we can do logical calculations through different operators: &&
(and), ||
(or), !
(not). For example, a XOR b is,
(!a && b) || (a && !b)
(4) Comparison Operators
Fundamental operations that are defined for each primitive type allow us to compare values.
- Operands: two expressions of the same type.
- Result: a value of type boolean.
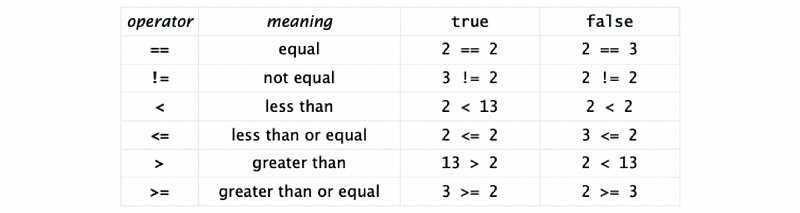
For example, if we want to find out whether a year is a leap year,
this will give us a boolean result.
6. Other Built-in Types
- short: integers from -2¹⁵ to 2¹⁵ -1
- long: integers from -2⁶³ to 2⁶³ -1
- float: real numbers
Note that the operators of these data types are the same as int or double.
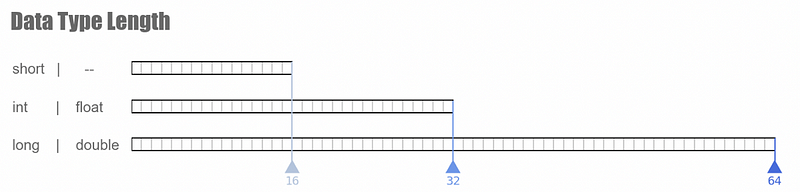
7. Excerpts From Java’s Math Library
- absolute value
double abs(double a)
- maximum value
double max(double a, double b)
- minimum value
double min(double a, double b)
- sin function: theta should be radians
double sin(double theta)
- cosine function: theta should be radians
double cos(double theta)
- tangent function: theta should be radians
double tan(double theta)
Note that if we want to convert the parameter, we can use toDegrees()
and toRadians()
to convert.
- exponential ( e^a )
double exp(double a)
- natural log ( ln(a) )
double log(double a)
- raise a to the b-th power
double pow(double a, double b)
- round to the nearest integer
long round(double a)
- random number in [0, 1)
double random()
- square root
double sqrt(double a)
- value of e
double E
- value of π
double PI
Now, let’s see an example,
this will give us the roots of a quadratic equation.
8. Compile Error
Sometimes, if we have some serious bugs, then the IED can not compile the program for us. Suppose we have the following code,
We can not compile this because we can not multiply a string with an integer.
9. Type Conversion
(1) Three Ways of Type Conversion
There are three ways of type conversion,
- Explicitly defined for function call
To change string to int, we can use the system call Integer.parseInt(),
- Automatic
To change int to string, we can directly use the + operator,
This also happens when we make numeric types match if no loss of precision.
- Cast
This can be used for: (1) small integers can be short, int, or long; (2) double values can be truncated to int values. For example,
(int) 2.71828 # 2
11 * (int) 0.25 # 0
(2) The Worst Type Conversion
A misused type conversion could cost a lot. The most famous case is the very first launch of Ariane 5. The fault is because the BH value (which is used to determine whether to point up or down) is stored by a 64-bit floating variable, which was perfectly adequate. The problem occurred when the software attempted to stuff this 64-bit variable, which can represent billions of potential values, into a 16-bit integer, which can only represent 65,535 potential values. Finally, the rocket crashed and this led to a public inquiry.
(3) Golden Rules For Type Conversion
- Avoid using them in the first place.
- Or live with a well-defined result.
- Or CRASH!