Java Programming Lab 1 | Conditionals and Loops
Java Programming Lab 1 | Conditionals and Loops
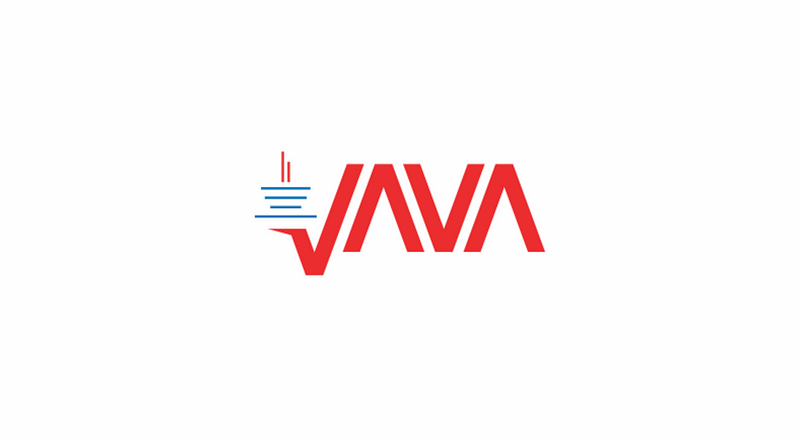
- Bits
Write a program Bits.java that takes an integer command-line argument n and uses a while loop to compute the number of times you need to divide n by 2 until it is strictly less than 1. Print the error message “Illegal input” if n is negative.
2. Noon snooze
Write a program NoonSnooze.java that takes an integer command-line argument snooze and prints the time of day (using a 12-hour clock) that is snooze minutes after 12:00pm (noon).
3. A drone’s flight
A drone begins flying aimlessly, starting at Nassau Hall. At each time step, the drone flies one meter in a random direction, either north, east, south, or west, with probability 25%. How far will the drone be from Nassau Hall after n steps? This process is known as a two-dimensional random walk.
(a) Write a program RandomWalker.java that takes an integer command-line argument n and simulates the motion of a random walk for n steps. Print the location at each step (including the starting point), treating the starting point as the origin (0, 0). Also, print the square of the final Euclidean distance from the origin.
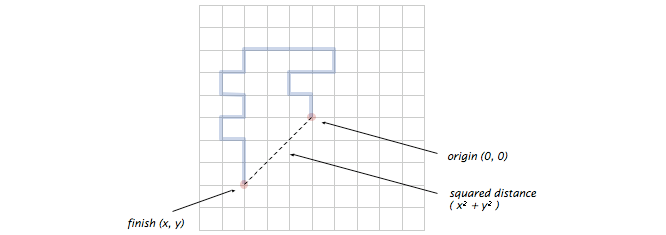
(b) Write a program RandomWalkers.java that takes two integer command-line arguments n and trials. In each of the trials independent experiments, simulate a random walk of n steps and compute the squared distance. Output the mean squared distance (the average of the trials squared distances).
4. Dice and the Gaussian distribution
Write a program RollDice.java that takes an integer command-line argument n, and rolls 10 fair six-sided dice, n times. Use an integer array to tabulate the number of times each possible total (between 10 and 60) occurs. Then print a text histogram of the results, as illustrated below.
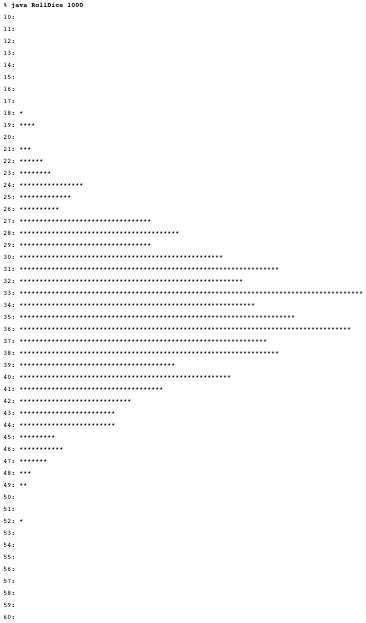