Java Programming 4 | Refresher
Java Programming 4 | Refresher
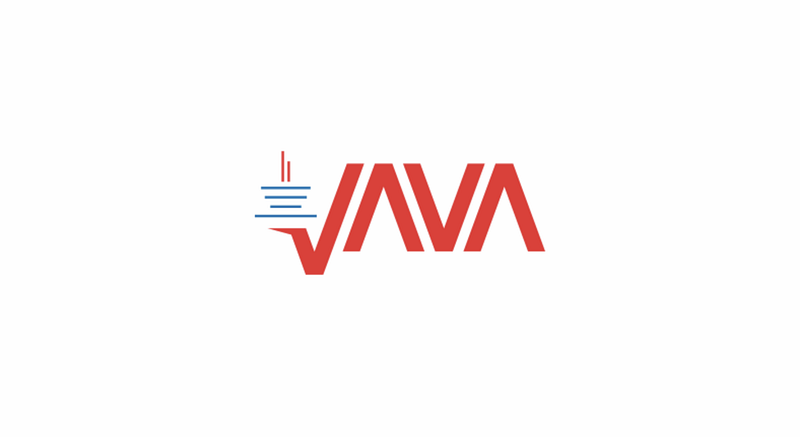
It’s been a long time since the last time when we talked about this series on java programming. Therefore, we have to review it a little bit before we continue. You can check yourself by answering the following questions.
Question 1. How to run the following code with the command lines?
public class Hello{
public static void main(String[] args){
System.out.println("Hello, world!");
}
}
Answer:
The file name must be the same as the public class name. So,
$ java Hello.java
Or we can run this code by,
$ javac Hello.java
$ java Hello
Question 2. What is the output of the following code?
public class Hello{
public static void main(String[] args){
System.out.println("Hello, world!\n");
System.out.println("Hello, world!");
}
}
Answer:
Hello, world!
Hello, world!
- What should be put in the blank if we want to print
Luck
?
public class PrintStr{
public static ___1___ main(String[] args){
____2____ a = "Luck";
System.out.println(a);
}
}
Answer:
1. void
2. String
Question 3. Suppose we have the following code,
public class PrintStr{
public static void main(String[] args){
System.out.println(args);
}
}
If we run the code above with,
$ java PrintArgs.java test
Can we print this test
argument? If not, how can we revise this code?
Answer:
No. The code should be,
public class PrintStr{
public static void main(String[] args){
System.out.println(args[0]);
}
}
Question 4. Suppose we have the following two scripts,
public class UseName{
public static void main(String[] args){
Name a = ___1___ Name("Adam");
System.out.println(a.name);
}
}
And,
public class Name{
String name;
Name(String a) {
____2____ = a;
}
}
Fill in the blank, and answer how to compile UseName.java
so it can use the class Name
?
Answer:
1. new
2. this.name
The file should be compiled by,
$ javacUseName.java
$ javaUseName
Question 5. Suppose we have a program and we run this program by command,
$ java TwoSum 1 2
If the raw code of this program is as follows,
public class TwoSum{
public static void main(String[] args) {
______1_____ i = ________2_______(args[0]);
______3_____ j = ________4_______(args[1]);
System.out.println(i + j);
}
}
What should we fill in the blanks if we want to print 12
? And what should we fill in the blanks if we want to print 3
? (Keep blank if you think there’s no need to fill in anything)
Answer:
To print 12,
1. String
2.
3. String
4.
To print 3,
1. int
2. Integer.parseInt(args[0])
3. int
4. Integer.parseInt(args[1])
Question 6. What should be the output of the following code?
public class TwoSum{
public static void main(String[] args) {
int i = Integer.parseInt(args[0]);
System.out.println(i + args[1]);
}
}
Answer:
12, because integers will be automatically converted to strings if they are added with strings.
Question 7. Suppose we have a program that can calculate the N (N is an integer) power of 2. Fill in the following blanks.
public class Power{
public static void main(String[] args) {
int c = ___1___ _____2_____(2, _____3_____);
System.out.println(c);
}
}
Answer:
1. (int)
2. Math.pow
3. Integer.parseInt(args[0])
Question 7. Suppose we have a program that can calculate the N (N can be double) power of 2. Fill in the following blanks.
public class Power{
public static void main(String[] args) {
double c = _____1_____(2, _____2_____);
System.out.println(c);
}
}
Answer:
1. Math.pow
2. Double.parseDouble(args[0])
Question 8. Suppose we have the following program. We would like to use it for printing Hello, World!
for N times (N must be an integer). We have to print Illegal Argument Exception
if N is smaller than 0. Fill in the following blanks,
public class CondAndLoops{
public static void main(String[] args) {
int i = Integer.parseInt(args[0]);
if (____1____) {
for (int j = 0; ____2____; j++) {
System.out.println("Hello, World!");
}
} else {
System.out.println("Illegal Argument Exception.");
}
}
}
Answer:
1. i >= 0
2. j < i
Question 9. Suppose we have an algorithm that will conduct element-wise addition on two arrays a
and b
. The result should be stored in array c
. Fill in the following blanks to complete this program.
public class Array {
public static void main(String[] args) {
___1___ a = {2, 3, 5};
___2___ b = {1, 4, 7};
___3___ c = ___4___ int[3];
for (int i = 0; ___5___; i++) {
___6___ = a[i] + b[i];
}
System.out.println(c[0]);
System.out.println(c[1]);
System.out.println(c[2]);
}
}
Answer:
1. int[]
2. int[]
3. int[]
4. new
5. i < 3
6. c[i]
Question 10. Suppose your friend Adam would like to collect free toys in 6 different colors. Each time he order a $12 meal at pizzeria, he will get one of them by random. If there is equal opportunity to get each color in one trial, what is the expected amount of money he has to spend in order to have the whole collection of 6 colors? (Euler’s constant = 0.57721)
Answer: He is expected to spend 162 dollars.
1. Mlog(M + 0.57721M) = 13.48450
2. 13.48450 * 12 = 162
Before you continue, download the files from here.
Coding 1. Simple HelloWorld
Your first task is to write the HelloWorld program. In IntelliJ, select File > New > Java Class. Name the file HelloWorld then type in everyone’s first program: HelloWorld.java
.
You will use the javac-introcs and java-introcs commands (instead of the default javac and java commands) to compile and execute your programs. These versions provide access to our course libraries.
$ javac-introcs HelloWorld.java
$ java-introcs HelloWorld
Answer:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Coding 2. Hi Four
This exercise demonstrates the use of the String data type and command-line arguments. Write a program HiFour.java
that takes four first names as command-line arguments and prints a proper sentence with the names in the reverse of the order given.
Examples:
> java-introcs HiFour Alice Bob Carol Dave
Hi Dave, Carol, Bob, and Alice.
> java-introcs HiFour Alejandro Bahati Chandra Deshi
Hi Deshi, Chandra, Bahati, and Alejandro.
Answer:
public class HiFour {
public static void main(String[] args) {
System.out.println("Hi " + args[3] + ", " + args[2] + ", " + args[1] + ", and " + args[0] + ".");
}
}
Exceptions:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 0 means that we forget to type a command-line argument when we executed the program.
Coding 3. Ordered
This exercise demonstrates the use of the int
and boolean
data types. Write a program Ordered.java
that takes three integer command-line arguments, x
, y
, and z
. Define a boolean variable whose value is true
if the three values are either in strictly ascending order (x < y < z
) or in strictly descending order (x > y > z
), and false
otherwise. Then, print this boolean value. You must not use any if
statements.
Examples:
> java-introcs Ordered 10 17 49
true
> java-introcs Ordered 49 17 10
true
> java-introcs Ordered 10 49 17
false
Answer:
public class Ordered {
public static void main(String[] args) {
int x = Integer.parseInt(args[0]);
int y = Integer.parseInt(args[1]);
int z = Integer.parseInt(args[2]);
boolean a = (x - y)*(y - z) > 0;
System.out.println(a);
}
}
Coding 4. Deluxe Ordered
This program, DeluxeOrdered.java
, performs the same operation as Ordered.java
: takes three int command-line arguments, x
, y
, and z
, and prints true if the three values are either in strictly ascending order (x < y < z
) or in strictly descending order (x > y > z
) and false otherwise. The challenge is to implement DeluxeOrdered.java
without using the four comparison operators <
, <=
, >
, >=
and if
statements.
Answer:
public class DeluxeOrdered {
public static void main(String[] args) {
int x = Integer.parseInt(args[0]);
int y = Integer.parseInt(args[1]);
int z = Integer.parseInt(args[2]);
int a = (x - y) >> 31;
int b = (y - z) >> 31;
boolean c = (a ^ b) == 0;
System.out.println(c);
}
}
Coding 5. Great Circle
This exercise demonstrates the use of the double data type and Java’s Math library. The great circle distance is the shortest distance between two points on the surface of a sphere if you are constrained to travel along the surface. Write a program GreatCircle.java that takes four double command-line arguments x1, y1, x2, and y2 (the latitude and longitude, in degrees, of two points on the surface of the earth) and prints the great circle distance (in nautical miles) between them. Use the following formula, which is derived from the spherical law of cosines:

This formula uses degrees, whereas Java’s trigonometric functions use radians. Use Math.toRadians()
and Math.toDegrees()
to convert between the two. For reference, a nautical mile (approximately 1.151 regular miles) is 1/60 of a degree of an arc along a meridian of the Earth, and this is the reason for the 60 in the formula.
Examples:
> java-introcs GreatCircle 40.35 74.65 48.87 -2.33 // Princeton to Paris
3185.1779271158425 nautical miles
> java-introcs GreatCircle 48.87 -2.33 40.35 74.65 // Paris to Princeton
3185.1779271158425 nautical miles
Answer:
public class GreatCircle {
public static void main(String[] args) {
double x1 = Math.toRadians(Double.parseDouble(args[0]));
double y1 = Math.toRadians(Double.parseDouble(args[1]));
double x2 = Math.toRadians(Double.parseDouble(args[2]));
double y2 = Math.toRadians(Double.parseDouble(args[3]));
double distance = 60 * Math.toDegrees(Math.acos(Math.sin(x1)*Math.sin(x2) + Math.cos(x1)*Math.cos(x2)*Math.cos(y1-y2)));
System.out.println(distance + " nautical miles");
}
}
Coding 6. RGB to CMYK
This exercise demonstrates the use of type conversions. Several different formats are used to represent color. For example, the primary format for LCD displays, digital cameras, and web pages — known as the RGB format — specifies the level of red (R), green (G), and blue (B) on an integer scale from 0 to 255 inclusive. The primary format for publishing books and magazines — known as the CMYK format — specifies the level of cyan (C), magenta (M), yellow (Y), and black (K) on a real scale from 0.0 to 1.0 inclusive.
Write a program RGBtoCMYK.java
that converts from RGB format to CMYK format. Your program must take three integer command-line arguments, red, green, and blue; print the RGB values; then print the equivalent CMYK values using the following mathematical formulas.
You may not use if
statements on this assignment, but you may assume that the command-line arguments are not all simultaneously zero.
Recall that Math.max(x, y) returns the maximum of x and y.
For full credit, your programs must not only work correctly for all valid inputs, but they should be easy to read.

CMYK is a subtractive color space, because its primary colors are subtracted from white light to produce the resulting color: cyan absorbs red, magenta absorbs green, and yellow absorbs blue.
Examples:
// indigo
> java-introcs RGBtoCMYK 75 0 130
red = 75
green = 0
blue = 130
cyan = 0.423076923076923
magenta = 1.0
yellow = 0.0
black = 0.4901960784313726
// Princeton orange
> java-introcs RGBtoCMYK 255 143 0
red = 255
green = 143
blue = 0
cyan = 0.0
magenta = 0.4392156862745098
yellow = 1.0
black = 0.0
Answer:
public class RGBtoCMYK {
public static void main(String[] args) {
double red = Double.parseDouble(args[0]);
double green = Double.parseDouble(args[1]);
double blue = Double.parseDouble(args[2]);
double white = Math.max(red/255, green/255);
white = Math.max(white, blue/255);
double cyan = (white - red/255) / white;
double magenta = (white - green/255) / white;
double yellow = (white - blue/255) / white;
double black = 1 - white;
System.out.println("red = " + (int) red);
System.out.println("green = " + (int) green);
System.out.println("blue = " + (int) blue);
System.out.println("cyan = " + cyan);
System.out.println("magenta = " + magenta);
System.out.println("yellow = " + yellow);
System.out.println("black = " + black);
}
}
Coding 7. Deluxe RGB to CMYK
This program, DeluxeRGBtoCMYK.java
, performs the same operation as RGBtoCMYK.java: converts RGB values to CMYK values. The challenge is to implement RGBtoCMYK.java
without using Math.max
, Math.min
, or if
statements.
Answer:
public class DeluxeRGBtoCMYK {
public static void main(String[] args) {
double red = Double.parseDouble(args[0]);
double green = Double.parseDouble(args[1]);
double blue = Double.parseDouble(args[2]);
double white = red/255 > green/255 ? red/255 : green/255;
white = white > blue/255 ? white : blue/255;
double cyan = (white - red/255) / white;
double magenta = (white - green/255) / white;
double yellow = (white - blue/255) / white;
double black = 1 - white;
System.out.println("red = " + (int) red);
System.out.println("green = " + (int) green);
System.out.println("blue = " + (int) blue);
System.out.println("cyan = " + cyan);
System.out.println("magenta = " + magenta);
System.out.println("yellow = " + yellow);
System.out.println("black = " + black);
}
}
Coding 8. Bits
Write a program Bits.java
that takes an integer command-line argument n and uses a while loop to compute the number of times you need to divide n by 2 until it is strictly less than 1. Print the error message “Illegal input” if n is negative.
Example:
> java-introcs Bits 0
0
> java-introcs Bits 1
1
> java-introcs Bits 2
2
> java-introcs Bits 4
3
> java-introcs Bits 8
4
> java-introcs Bits 16
5
> java-introcs Bits 1000
10
> java-introcs Bits -23
Illegal input
Answer:
public class Bits {
public static void main(String[] args) {
double n = Double.parseDouble(args[0]);
if (n < 0) {
System.out.println("Illegal input");
return;
}
int i = 0;
while (n >= 1) {
n = n / 2;
i++;
}
System.out.println(i);
}
}
Coding 9. Noon Snooze
Write a program NoonSnooze.java
that takes an integer command-line argument representing the number of minutes, snooze, that have elapsed since 12:00pm
(noon) and prints the resulting time. Assume a 12-hour clock (with am
and pm
). You must not use loops. You may assume that the value of snooze is a non-negative integer.
Example:
> java-introcs NoonSnooze 50
12:50pm
> java-introcs NoonSnooze 100
1:40pm
> java-introcs NoonSnooze 721
12:01am
> java-introcs NoonSnooze 11111
5:11am
Answer:
public class NoonSnooze {
public static void main(String[] args) {
int hour = 12;
int mins = 00;
int minplus = Integer.parseInt(args[0]);
hour = (hour + minplus / 60) % 12;
if (hour == 0) hour = 12;
mins = minplus % 60;
if (minplus / 60 / 12 % 2 == 0) {
System.out.println(hour + ":" + String.format("%02d", mins) + "pm");
} else {
System.out.println(hour + ":" + String.format("%02d", mins) + "am");
}
}
}
Coding 10. Deluxe Noon Snooze
This program, DeluxeNoonSnooze.java, performs the same operation as NoonSnooze.java: takes a command-line argument, snooze, of the elapsed minutes since 12:00pm
(noon) and prints the resulting time.
The challenge is to implement DeluxeNoonSnooze.java without using any if
statements. Additionally, it must be able to handle negative snooze values.
Answer:
public class DeluxeNoonSnooze {
public static void main(String[] args) {
int hour = 12;
int mins = 00;
int minplus = Integer.parseInt(args[0]);
String meridiem;
hour = (hour + minplus / 60) % 12;
hour = (hour == 0) ? 12 : hour;
mins = minplus % 60;
meridiem = ((minplus >= 0) && (minplus / 60 / 12 % 2 == 0)) ? "pm" : "am";
meridiem = ((minplus < 0) && (minplus / 60 / 12 % 2 == 0)) ? "am" : "pm";
// handle negative
hour = (mins < 0) ? hour - 1: hour;
mins = (mins < 0) ? 60 + mins : mins;
hour = (hour < 0) ? 12 + hour : hour;
System.out.println(hour + ":" + String.format("%02d", mins) + meridiem);
}
}
Coding 11. Random Walker
A drone begins flying aimlessly, starting at Nassau Hall (0, 0)
. At each time step, the drone flies one meter in a random direction, either north, east, south, or west, with a probability of 25%. How far will the drone be from Nassau Hall after n
steps? This process is known as a two-dimensional random walk.
Write a program RandomWalker.java
that takes an integer command-line argument n and simulates the motion of a random walk for n
steps. Print the location at each step (including the starting point), treating the starting point as the origin (0, 0)
. Finally, print the square of the final Euclidean distance from the origin.
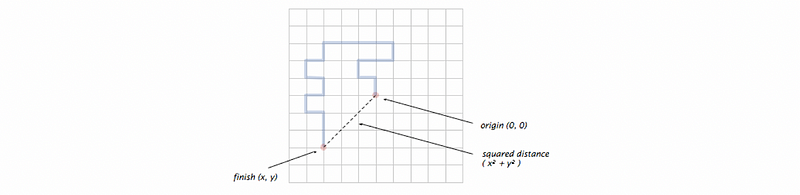
Examples:
> java-introcs RandomWalker 10
(0, 0)
(0, -1)
(0, 0)
(0, 1)
(0, 2)
(-1, 2)
(-2, 2)
(-2, 1)
(-1, 1)
(-2, 1)
(-3, 1)
squared distance = 10
> java-introcs RandomWalker 20
(0, 0)
(0, 1)
(-1, 1)
(-1, 2)
(0, 2)
(1, 2)
(1, 3)
(0, 3)
(-1, 3)
(-2, 3)
(-3, 3)
(-3, 2)
(-4, 2)
(-4, 1)
(-3, 1)
(-3, 0)
(-4, 0)
(-4, -1)
(-3, -1)
(-3, -2)
(-3, -3)
squared distance = 18
Answer:
public class RandomWalker {
public static void main(String[] args) {
int x = 0;
int y = 0;
int n = Integer.parseInt(args[0]);
while (n > 0) {
int direction = (int) (Math.random() * 4);
if (direction == 0) {
x--;
n--;
} else if (direction == 1) {
y--;
n--;
} else if (direction == 2) {
x++;
n--;
} else {
y++;
n--;
}
System.out.println("(" + x + ", " + y + ")");
}
int sdist = x*x + y*y;
System.out.println("squared distance = " + sdist);
}
}
Coding 12. Random Walkers
Write a program RandomWalkers.java
that takes two integer command-line arguments n
and trials
. In each of trials independent experiments, simulate a random walk of n steps and compute the squared distance. Output the mean squared distance (the average of the trials squared distances).
Answer:
public class RandomWalkers {
public static void main(String[] args) {
double totalSquaredDistance = 0;
double trials = Integer.parseInt(args[1]);
for (int i = 0; i < trials; i++) {
double x = 0;
double y = 0;
int n = Integer.parseInt(args[0]);
while (n > 0) {
int direction = (int) (Math.random() * 4);
if (direction == 0) {
x--;
n--;
} else if (direction == 1) {
y--;
n--;
} else if (direction == 2) {
x++;
n--;
} else {
y++;
n--;
}
}
totalSquaredDistance = totalSquaredDistance + x*x + y*y;
}
System.out.println("mean squared distance = " + totalSquaredDistance/trials);
}
}