Relational Database Lab 2 | CRUD and Select Operations Practice, Variables, Case, and Pivot…
Relational Database Lab 2 | CRUD and Select Operations Practice, Variables, Case, and Pivot Statement
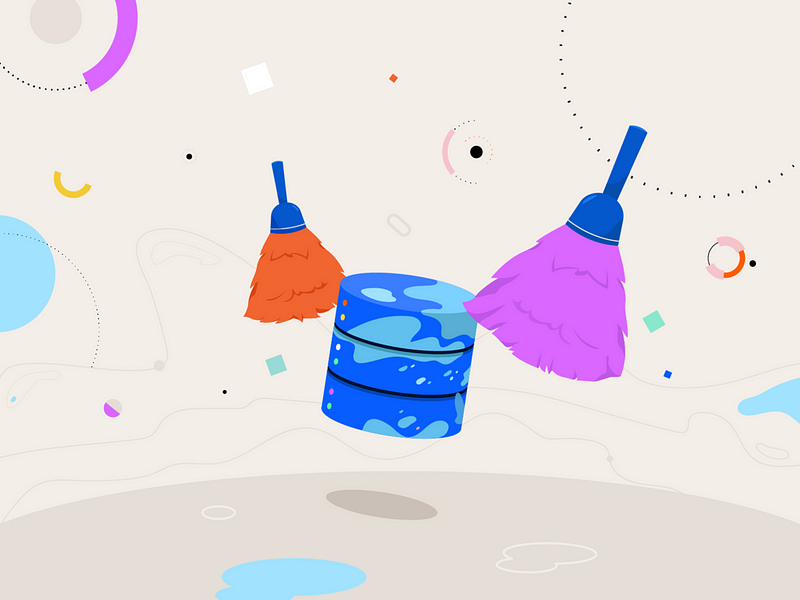
Some of the practice problems are from https://www.hackerrank.com/.
1. CRUD Operations
(1) Create a Database
Create a database named PRACTICE.
(2) CREATE Table
Create a table named CITY and the CITY table is described as follows:
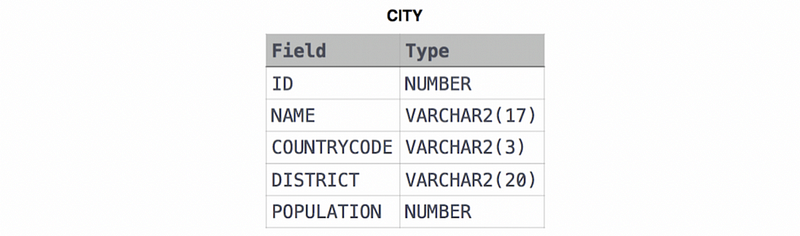
Note that all the values in this table are NOT NULL.
(3) INSERT Values
Add the following data to the CITY table:
6 Rotterdam NLD Zuid-Holland 593321
3878 Scottsdale USA Arizona 202705
3965 Corona USA California 124966
3973 Concord USA California 121780
3977 Cedar Rapids USA Iowa 120758
3982 Coral Springs USA Florida 117549
4054 Fairfield USA California 92256
4058 Boulder USA Colorado 91238
4061 Fall River USA Massachusetts 90555
(4) UPDATE the Population in Rotterdam
There is a change in the population in Rotterdam to 582571, update this value in the CITY table.
(5) DELETE the City of Rotterdam
Delete the city named Rotterdam in the CITY table.
(6) SELECT the result
Output the content of the CITY table.
2. SELECT Operation
(1) Practice #1
Query all columns for all American cities in the CITY table with populations larger than 100000
. The CountryCode for America is USA
. The CITY table is described as follows (Note that the values in this table are not the same as what we have put inside above):
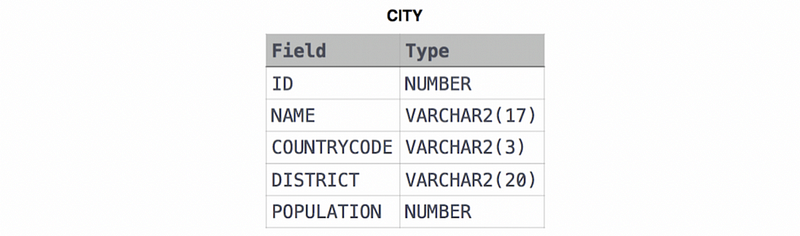
(2) Practice #2
For the same table of practice #1, query all columns (attributes) for every row in the CITY table.
(3) Practice #3
For the same table of practice #1, query all columns for a city in CITY with the ID 1661
.
(4) Practice #4
For the same table of practice #1, query all attributes of every Japanese city in the CITY table. The COUNTRYCODE for Japan is JPN
.
(5) Practice #5
For the same table of practice #1, query the names of all the Japanese cities in the CITY table. The COUNTRYCODE for Japan is JPN
.
(6) Practice #6
Query a list of CITY and STATE from the STATION table. The STATION table is described as follows where LAT_N is the northern latitude and LONG_W is the western longitude.
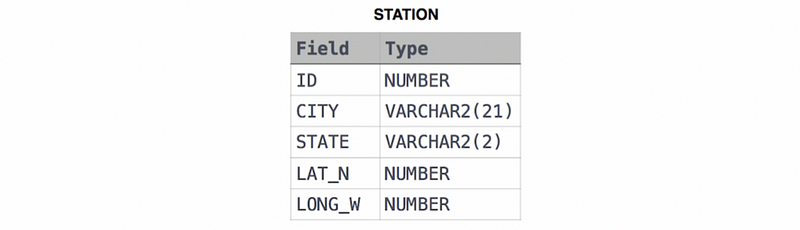
(7) Practice #7
For the same table of practice #6, query a list of CITY names from STATION for cities that have an even ID number. Print the results in any order, but exclude duplicates from the answer.
(8) Practice #8
For the same table of practice #6, find the difference between the total number of CITY entries in the table and the number of distinct CITY entries in the table.
For example, if there are three records in the table with CITY values ‘New York’, ‘New York’, ‘Bengaluru’, there are 2 different city names: ‘New York’ and ‘Bengaluru’. The query returns 1.
(9) Practice #9
For the same table of practice #6, query the two cities in STATION with the shortest and longest CITY names, as well as their respective lengths (i.e.: number of characters in the name). If there is more than one smallest or largest city, choose the one that comes first when ordered alphabetically.
Sample Input
For example, CITY has four entries: DEF, ABC, PQRS, and WXY.
Sample Output
ABC 3
PQRS 4
(10) Practice #10
For the same table of practice #6, query the list of CITY names starting with vowels (i.e., a
, e
, i
, o
, or u
) from STATION. Your result cannot contain duplicates.
(11) Practice #11
For the same table of practice #6, query the list of CITY names ending with vowels (a, e, i, o, u) from STATION. Your result cannot contain duplicates.
(12) Practice #12
For the same table of practice #6, query the list of CITY names from STATION which have vowels (i.e., a, e, i, o, and u) as both their first and last characters. Your result cannot contain duplicates.
(13) Practice #13
For the same table of practice #6, query the list of CITY names from STATION that do not start with vowels. Your result cannot contain duplicates.
(14) Practice #14
For the same table of practice #6, query the list of CITY names from STATION that either does not start with vowels or do not end with vowels. Your result cannot contain duplicates.
(15) Practice #15
Query the Name of any student in STUDENTS who scored higher than 75 Marks. Order your output by the last three characters of each name. If two or more students both have names ending in the same last three characters (i.e.: Bobby, Robby, etc.), secondary sort them by ascending ID. The STUDENTS table is described as follows and The Name column only contains uppercase (A
-Z
) and lowercase (a
-z
) letters.
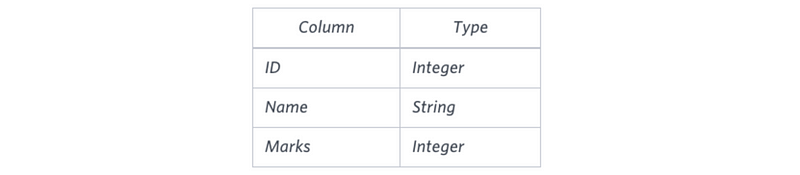
(16) Practice #16
Write a query that prints a list of employee names (i.e.: the name attribute) from the Employee table in alphabetical order. The Employee table containing employee data for a company is described as follows:
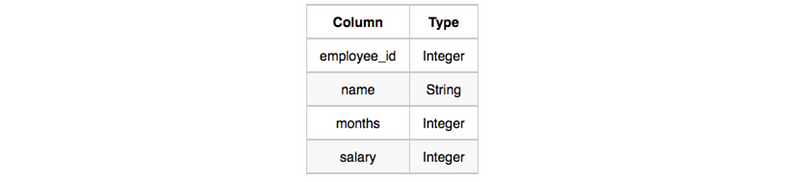
where employee_id is an employee’s ID number, name is their name, months is the total number of months they’ve been working for the company, and salary is their monthly salary.
(17) Practice #17
For the same table of practice #16, write a query that prints a list of employee names (i.e.: the name attribute) for employees in Employee having a salary greater than $2000 per month who have been employees for less than 10 months. Sort your result by ascending employee_id.
(18) Practice #18
For the same table of practice #1, query the total population of all cities in CITY where District is California.
(19) Practice #19
For the same table of practice #1, query the average population of all cities in CITY where District is California.
(20) Practice #20
For the same table of practice #1, query the average population for all cities in CITY, rounded down to the nearest integer.
(21) Practice #21
For the same table of practice #1, query the difference between the maximum and minimum populations in CITY.
(22) Practice #22
Query an alphabetically ordered list of all names in OCCUPATIONS, immediately followed by the first letter of each profession as a parenthetical (i.e.: enclosed in parentheses). For example: AnActorName(A)
, ADoctorName(D)
, AProfessorName(P)
, and ASingerName(S)
.
There will be at least two entries in the table for each type of occupation. The OCCUPATIONS table is described as follows and occupation will only contain one of the following values: Doctor, Professor, Singer, or Actor.
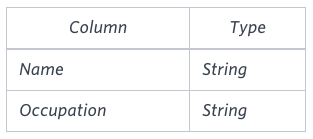
(23) Practice #23
For the same table of practice #22, query the number of occurrences of each occupation in OCCUPATIONS. Sort the occurrences in ascending order, and output them in the following format:
There are a total of [occupation_count] [occupation]s.
where [occupation_count]
is the number of occurrences of occupation in OCCUPATIONS and [occupation]
is the lowercase occupation name. If more than one Occupation has the same [occupation_count]
, they should be ordered alphabetically.
2. Variables, Case, and Pivot Statement
(1) Practice #24
Set the variables myName and myNum, and then print <myName> is <myNum> years old.
However, this is a SQL Server feature, and if we want to use variables in PostgreSql, we have to use the WITH operation,
(2) Practice #25
For the same table of practice #1, query a new column called citysize and when the population is larger than 150000, give an ‘L’ to this column, else if the population is between 100000 to 150000, give an ‘M’ to this column, and for the rest of the values, give an ‘S’.
We can also include the names of cities in the output,
We can make the output a new table named CITYSIZE,
Also, set the NAME column as our primary key,
(3) Practice #26
For the same table of practice #1, pivot the POPULATION column in CITY so that we can have a sum of the population in each district. We are assuming that you are probably using PostgreSQL and here’s an example to create this pivot,
(3) Practice #27
For the same table of practice #1, pivot the POPULATION column in CITY so that we can have a sum of the population in each district by countries. We are assuming that you are probably using PostgreSQL and here’s an example to create this pivot,