Software Development Process 5 | Object Orientated Programming and Unified Modeling Language
Software Development Process 5 | Object Orientated Programming and Unified Modeling Language
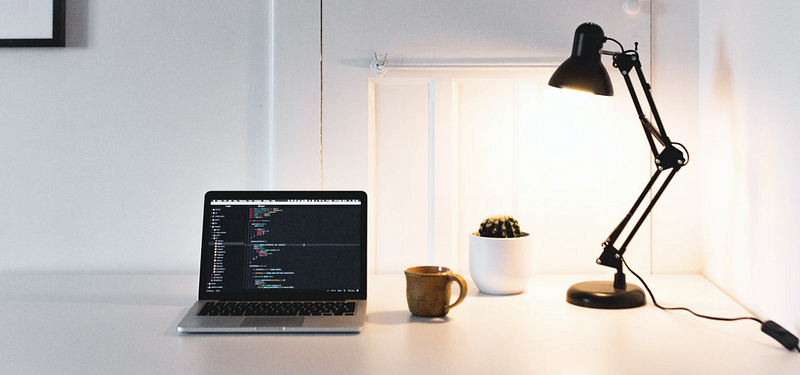
- Object Orientated
(1) The Definition of Object Orientation
Object orientation is to give precedence of data over function. This also allows for enforcing the very important concept of information hiding, which is the encapsulation and segregation of data behind well-defined and ideally stable interfaces in order to be able to hide the design and also implementation decisions.
(2) Reasons for Object Orientation
One of the main reasons is that it makes code more maintainable because the rest of the code or system doesn’t have to be concerned about how the implementation details or the design are defined. Therefore, any change that happens behind this wall doesn't concern or affect the rest of the system as long as you keep your interfaces consistent.
Another advantage of focusing on objects and encapsulating the information into cohesive entities is that it allows the reuse of object definitions by incremental refinement which is what we normally call inheritance. And inheritance is definitely a fundamental concept in object orientation.
(3) The Definition of Objects
An object is a computing unit organized around a collection of state or instance variables that define the state of the object. In addition, each object has associated with it a set of operations or methods that operate on such state.
(4) The Definition of Classes
A class is basically a template, a blueprint, if you wish, from which new objects (what we call instances of the class) can be created. The fact of having a blueprint for objects that allows us to create as many objects as we want can further reuse, and also contribute to make the code more readable, understandable, and therefore ultimately more maintainable.
(5) Benefits for Object Orientation
- Reduce Maintenance Cost: The first reason is that object orientation can help reduce long-term maintenance costs by limiting the effects of changes.
- Improve Development Process: Object orientation can also improve the developing process by favoring code and design reuse.
- Enforce Good Design: enforce good design principles such as the ones that we saw in encapsulation, information hiding, high cohesion, low coupling.
- Modular Coding Style: The modular coding style typical of object-oriented approaches can, and normally does, increase reuse.
- Increase Maintainability: Because they’re more modular, they’re more cohesive and decoupled, which contributes to the maintainability
- Increase Understandability: we can recognize in the systems, real-world entities that we are used to see and that we understand
(6) The History of Object Orientated Programming
- James Rumbaugh (1990s): developed an integrated approach to object modeling technique (OMT) with three main aspects, data, functions, and control
- Jacobson and Booch: OMT combined with contributions from several people evolved into what we call the Unified Modeling Language (UML). UML extends OMT by providing more diagrams and a broader view of a system from multiple perspectives.
(7) Object Orientated Analysis
Traditional analysis and design techniques were functionally oriented. What that means is that they concentrated on the functions to be performed, whereas the data upon which the functions operated were secondary to the functions themselves.
Conversely, object-oriented analysis is primarily concerned that with data objects. So we went from a functional-oriented view to a data-oriented view. What that means is that during the analysis phase, we define a system first in terms of the data types and their relationships, and the functions or methods are defined only later and associated with specific objects which are sets of data.
(8) Perform Object Orientated Analysis
The basic idea is to go from real-world objects to a set of requirements. We can describe this as a four-step process.
- Obtain and prepare a textural description of the problem: obviously, we need to start with some description of the system that we need to build.
- Underline nouns as classes
- Underline adjectives as attributes of the classes
- Underline active verbs as operations or methods for the classes
2. Unified Modeling Language (UML)
(1) The Definition of UMLs
These are the diagrams that represent the static characteristics of the system that we need to model. This is in contrast with dynamic models which instead behaviors of the system that we need to model.
(2) The Class Diagram
The class diagram is a fundamental one in UML, which represents a static, structural view of the system, and it describes the classes and their structure, and the relationships among classes in the system. A class is represented as a rectangle with three parts,
- Class name: singular nouns starting with a capital letter
- Attributes: title and its initial value
- Operations: name, inputs (arguments list), with a result type (also called the signatures of operations)
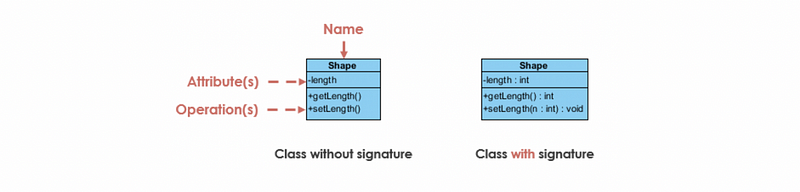
Something else you can notice in this representation is the fact that there is a minus sign before some attributes and a plus before others. The minus indicates that the attributes are private
to the class, so only instances of this class, roughly speaking, can access these attributes. The plus conversely indicates that this is a public
operation, so something that is visible outside the class.
(3) The Attributes Diagram
Attributes represent the structure of a class, the individual data items that compose the state of the class. Attributes may be found in one of three ways,
- By examining class definitions
- By studying the requirements
- By applying domain knowledge
Always keep in mind the domain knowledge is important for analysis, design gathering, and so on.
(4) The Operation Diagram
Operations represent the behavior of a class, and they may be found by examining interactions among entities in the description of your system. Now, there is one thing that we’re missing, a very important aspect of the class diagram which is the relationships between these classes.
(5) Class Diagram Relationships
Relationships describe interactions between classes or between objects in your system. We will describe three main types of relationships,
- Dependencies (X uses Y): dotted line with an arrow
- Associates / Aggregations (X has/contains a Y): solid line with diamond or nothing (the diamond end should be larger)
- Generations (X is a Y): solid line with an arrow

(6) Associates / Aggregations Adornments
However, in associates relationships or aggregations relationships, the solid line doesn’t really tell us much about the nature of the relationship. To clarify such nature we can use what we call adornments that we can add to associations to clarify their meaning.
In particular, we can add a label to an association and the label describes the nature of the relationship. We can also add a triangle to further clarify the direction of the relationship.
Another important adornment or limitation that we can put on an association, is multiplicity. Multiplicity defines the number of instances of one class that is related to one instance of the other class. We can define multiplicity at either end of the relationship.
(7) Creation Tips for Class Diagrams
- Understand the problem
- Choose good class names
- Concentrate on the WHAT: no need to think about HOW
- Start with a simple diagram
- Refine until you feel it is complete
(8) Component Diagram
A component diagram is a static view of components in a system and of their relationships. More precisely,
- Nodes: a node in a component diagram represents a component where a component consists of one or more classes with a well-defined Interface.
- Edges: conversely indicate relationships between the components.
Component diagrams can be used to represent architecture.
(9) Deployment Diagram
The deployment diagram provides a static deployment view of a system, and unlike the previous diagram, it is about the physical allocation of components to computational units.
Think, for example, of a client-server system in which you’ll have to define which components will go on the server and which component will go on the client. For deployment diagrams,
- Nodes: correspond to computation units such as a specific device.
- Edges: indicate communication between these units.
(10) The Definition of UML Behavioral Diagrams
We now discuss UMLs behavioral diagrams which are those diagrams that have to do with the behavior, the dynamic aspects of the system, rather than the static ones.
(11) UML Behavioral Diagrams: Use Case
One of the most fundamental behavioral diagrams is called the use case. A use case represents two main things,
- The sequence of interactions of outside entities (aka. actors) with the system that we’re modeling
- The system actions that yield an observable result of values to the actors.
So it’s the view of the system in which we look at the interaction between this system and the outside world. For example, they’re also called scenarios, scripts or user stories, but in the context of UML, we call them use cases.
(12) An Example of Use Case
Now, let’s look at use cases in a little more detail and start by defining exactly what an actor is. An actor represents an entity, which can be a human or a device that plays a role and interacts with the system. It is important to clarify,
- an entity can play more than one role
- more than one entity can play the same role
- actors can appear in more than one use case
(13) Documenting Use Cases
The behavior of a use case can be specified by describing its flow of events. And it is important to note that the flow of events should be described,
- From an actor’s point of view: so from the point of view of the external entity that is interacting with the system.
- How the use case starts and ends
- Normal flow of events: normal internation
- Alternate flow of events: multiple ways for accomplishing any tasks
- Excepted flow of events
(14) Two Ways of Documenting Use Cases
One more thing I want to mention, when we talk about documenting use cases, is the fact that the description of this information can be provided in two main ways, in an informal way or in a formal way.
- Informal description: we could just have a textual description of the flow of events in natural language.
- Formal/Structure description: we may use, for example, pre and post conditions, pseudo code to indicate the steps. We could also use the sequence diagrams.
(15) Role of Use Cases
Now, let’s discuss why they’re so important and what are the different roles that use cases can play.
- Requirement Elicitation: easier to describe what the system should do if we think about the system in terms of scenarios of usage
- Architectural Analysis: use cases are the starting point for the analysis of the architecture of the system that can help identify the main blocks of the system
- User Prioritization: users might want to prioritize some of the multiple actors in the system
- Planning: This becomes very important in many different software life cycles such as the unified software process and the agile development processes
- Testing: easily define test cases, even before writing the code, even before defining my system
(16) Creation Tips for Use Case Diagram
- Use name that communicates purpose: It should be clear what the use case refers to by just looking at the name of the use case.
- Define one atomic behavior per use case: try not to put more than one specific scenario into a use case because these will make the use cases easier to understand and better suited for their roles
- Define flow of events clearly: An outsider should be able to read the description of the flow of events and understand exactly how the system works or how that specific piece of functionality works.
- Provide only essential details: just provide enough details so that the use case is complete and understandable
- Factor common behaviors
- Factor variants
(17) UML Behavioral Diagrams: Sequence Diagram
A sequence diagram is an interaction diagram that emphasizes how objects communicate and the time ordering of the messages between objects. Basically, we can perform a sequence diagram by the following phases,
- place the objects that participate in the interaction at the top of the diagram along the x-axis
- add what is called the object lifeline (a vertical dashed line for each object, except for the outermost object for which it is a solid line)
- placing messages that these objects send and receive in order of increasing time, from top to bottom
(18) UML Behavioral Diagrams: State Transition Diagram
The state transition diagram is defined for each relevant class in the system and basically shows the possible life history of a given class or object. It means that,
- it describes the possible states of the class as defined by the values of the class attributes
- it describes the events that cause a transition from one state to another
- it describes the actions that result from a state change
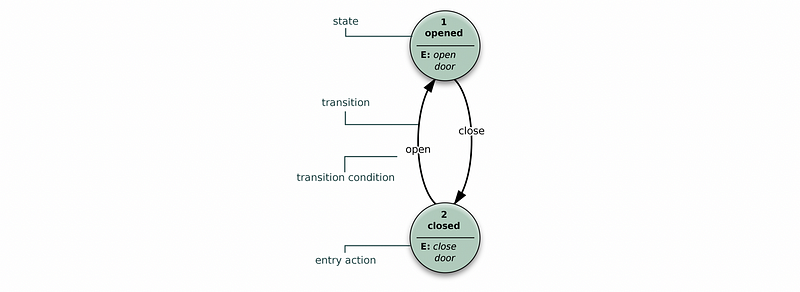
(19) Notations of State Transition Diagram
There are also some notations that are used to represent the state transition diagram.
- States are represented by ovals with a name
- Transitions marked by the event that triggers the transition. Notice that not all events will cause a state transition.
- Event may produce actions and they may also have attributes which are analogous to parameters in a method call and Boolean conditions that guard the state transition
event(attrs)[Conds]
STATE 1 ----------------------------> STATE 2
actions